We will view the functions which can be used for lists:
- list.append(item)
- list.extend(item)
- list.insert(index, item)
- list.remove(item)
- list.pop(index)
- list.clear()
- list.index(item)
- list.count(item)
- list.sort()
- list.reverse()
list.copy()
Let’s start with:
>>> animals = ['cat', 'dog', 'bee']
And for each method we will imagine if we had done this:
>>> animals = ['cat', 'dog', 'bee'] # Creating the dictionary again
(#) To add one item to the end of list:
list.append(item)
>>> animals.append('eagle')
>>> ['cat', 'dog', 'bee', 'eagle']
>>> couple = ['cow', 'bull'] >>> animals.append(couple) >>> animals ['cat', 'dog', 'bee', 'eagle', ['cow', 'bull']
(#) To concatenate an item to the end of list:
list.extend(item)
>>> animals.extend('eagle')
>>> ['cat', 'dog', 'bee', 'eagle']
>>> couple = ['cow', 'bull'] >>> animals.extend(couple) >>> animals ['cat', 'dog', 'bee', 'eagle', 'cow', 'bull']
(#) To insert one item at a given position, moving to the right which had:
list.insert(index, item)
>>> animals.insert(2, 'eagle')
>>> animals
['cat', 'dog', 'eagle', 'bee']
(#) To delete an item, the first found with whose element:
list.remove(item)
>>> animals.append('dog')
>>> animals
['cat', 'dog', 'bee', 'dog']
>>> animals.remove('dog')
>>> animals
['cat', 'bee', 'dog']
(#) To remove and catch an item:
list.pop(index)
>>> animals
['cat', 'dog', 'bee']
>>> animals.pop(1)
'dog'
>>> animals
['cat', 'bee']
If no index is specified, the method catches the last item in the list:
>>> animals
['cat', 'dog', 'bee']
>>> animals.pop()
'bee'
>>> animals
['cat', 'dog']
(#) To remove all items:
list.clear()
>>> animals.clear()
'dog'
>>> animals
[]
(#) To return the first index of an item:
list.index(item)
>>> animals.append('dog')
>>> animals['cat', 'dog', 'bee', 'dog']
>>> animals.index('dog')
1
(#) To return the times which an item appears:
list.count('dog')
>>> animals.append('dog')
>>> animals['cat', 'dog', 'bee', 'dog']
>>> animals.index('dog')
2
(#) To sort the items (e.g., alphabetically):
list.sort()
>>> animals
['cat', 'dog', 'bee']
>>> animals.sort()
['bee', 'cat', 'dog']
(#) To do the opossite than sort():
list.reverse()
>>> animals
['cat', 'dog', 'bee']
>>> animals.reverse()
>>> animals
['bee', 'dog', 'cat']
(#) To copy a list as independent:
list.copy()
>>> myanimals = animals
>>> myanimals
['cat', 'dog', 'bee']
>>> animals.append('cow')
>>> myanimals
['cat', 'dog', 'bee', 'cow']
>>> myanimals is animals
True
>>> animals.copy()
The copy() method does not run to me, however it is possible to use the list() function for example (read below, on more information):
>>> animals = ['cat', 'dog', 'bee'] >>> myanimals = list(animals) # new_list = list(old_list) >>> animals.append('cow') >>> myanimals ['cat', 'dog', 'bee'] >>> myanimals is animals False
More information:
- https://docs.python.org/3/tutorial/datastructures.html
- http://stackoverflow.com/questions/2612802/how-to-clone-or-copy-a-list-in-python
- https://docs.python.org/3.3/library/functions.html#func-list
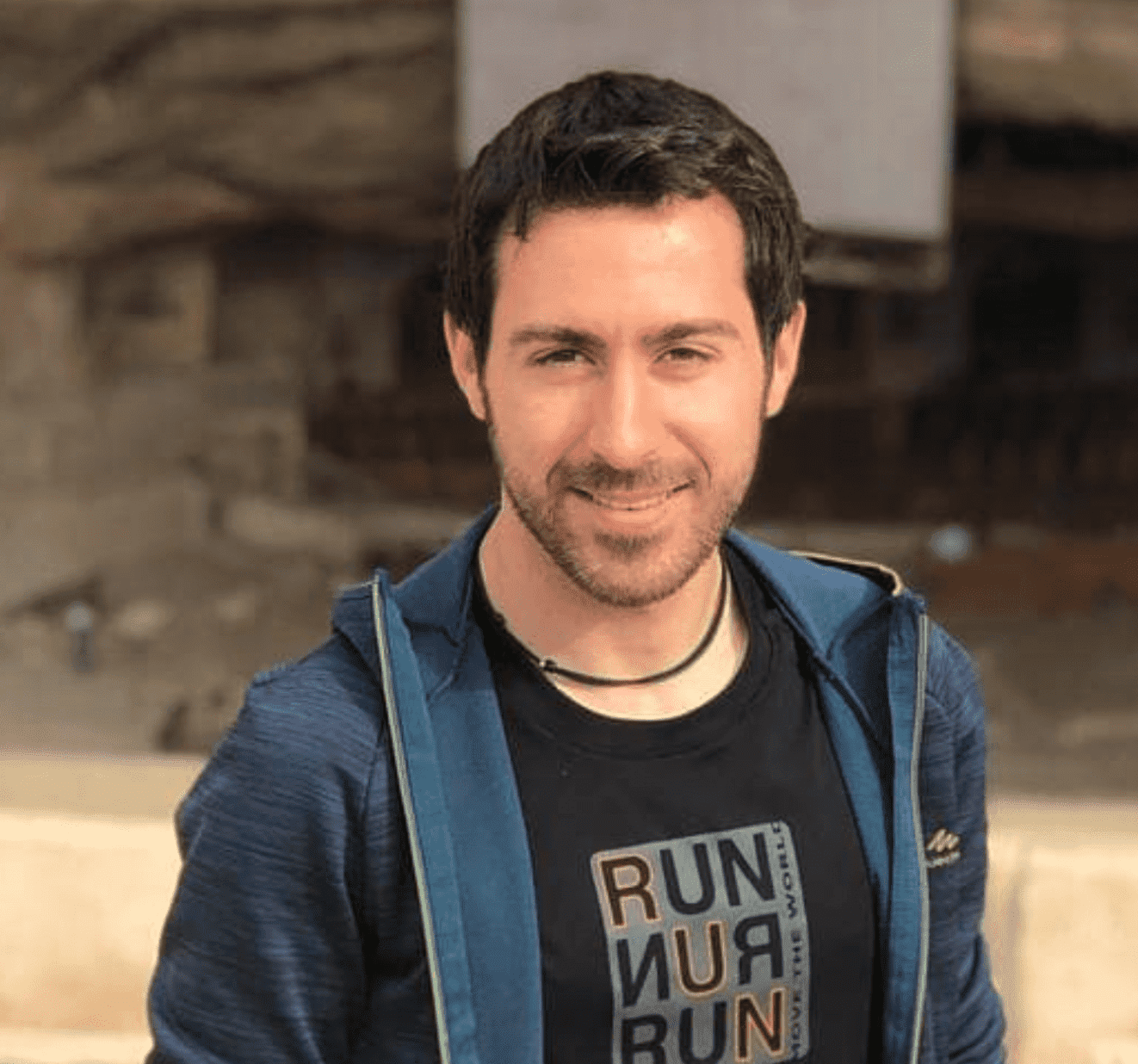
Reality builder, marketing passionate and entrepreneur.
Be First to Comment